In this article, I try to synthesize several (incomplete or inaccurate) articles I’ve found on the Internet to guide you through the process of creating a disk image you may use with the Bochs emulator. The steps described below are intended for a GNU/Linux system and some of them require super user privileges. Also, you need to have GRUB installed on your system.
1. Create a disk image. Here, I create a file named disk.img, containing 10080 blocks (each block being 512 bytes, this will create a file that’s about 5MB)
$ dd if=/dev/zero of=disk.img count=10080
2. Use FDISK to create a partition table on the image file:
$ fdisk disk.img
x -> Extra functionality
c 10 -> 10 cylinders
h 16 -> 16 heads
s 63 -> 63 sectors per track
r -> Return to main menu
n -> Create a new partition
p -> Primary
1 -> Partition #1
1 -> First cylinder
10 -> Last cylinder
a -> Set bootable flag
1 -> Partition number
w -> Write partition to disk
Note that you have to tell fdisk about the geometry of your disk, and that geometry has to match the size of the disk you created in step 1 (10 cylinders * 16 heads * 63 sectors per track = 10080 blocks). For an introduction on disk geometry, cylinders, heads and sectors, read this Wikipedia article.
Let’s take a quick look at the Partition Table in the Master Boot Record (See this Wikipedia article for a description of the structure of the partition table)
$ hexdump disk.img
The 16 bytes at offset 446 (0x1BE) are:
0180 0001 0f83 093f 003f 0000 2721 0000
Keep in mind that these values are stored using little-endian convention (see this Wikipedia article to find out more about the meaning of endianness in computer science). Also see this article to find out how the CHS values are computed.
Offset |
Description |
0×00 |
0×80 means that this partition is bootable |
0×01 |
0×000101 is the CHS address of the first sector in the partition: S = 0×01 = 1 H = 0×01 = 1 C = 0×00 = 0 |
0×04 |
0×83 is the type of the partition (Linux native here) |
0×05 |
0x093f0f is the CHS address of the last sector in the partition: S = 0x3F = 63 H = 0x0F = 15 C = 0×09 = 9 |
0×08 |
0x0000003f (63) is the logical block address of the first sector in the partition |
0x0C |
0×00002721 (10017 = 10080 - 63) is the size of the partition, in number of 512 byte blocks |
3. Setup the loopback device. In order to do this, you need to calculate the offset (in bytes) of the first sector of your single partition. Use the following command:
$ fdisk -l -u disk.img
Device Boot Start End Blocks Id System
disk.img1 * 63 10079 5008+ 83 Linux
This tells us that our single partition starts at the 63rd block. Hence our offset is 63 * 512 = 32256.
Finally, type:
$ losetup -o 32256 /dev/loop0 disk.img
4. Format the disk (EXT2FS)
$ mkfs.ext2 /dev/loop0
5. Mount the disk:
$ mount -o loop /dev/loop0 /mnt
6. Now, let’s install GRUB. Start by copying the necessary GRUB files:
$ mkdir -p /mnt/boot/grub
$ cp /boot/grub/stage1 /boot/grub/stage2 /mnt/boot/grub/
$ vi /mnt/boot/grub/grub.conf
title=MyKernel
root (hd0,0)
kernel /mykernel
7. Unmount the device:
$ umount /mnt
8. Detach the loopback device:
$ losetup -d /dev/loop0
9. Finish up the GRUB installation:
$ grub --device-map=/dev/null
device (hd0) disk.img
geometry (hd0) 10 16 63
root (hd0,0)
setup (hd0)
quit
10. Setup your .bochsrc file in the same directory as your disk image:
megs: 32
romimage: file=/usr/local/share/bochs/BIOS-bochs-latest, address=0xf0000
vgaromimage: file=/usr/local/share/bochs/VGABIOS-elpin-2.40
ata0-master: type=disk, path="disk.img", mode=flat, cylinders=10, heads=16, spt=63
cpu: count=1, ips=15000000
mouse: enabled=0
log: out.bochs
boot: disk
That’s it. Start Bochs and the GRUB interface should appear:
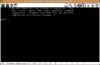